Fetch Follows
This section covers how to fetch follows on the Subsocial blockchain.
Check if a user is following a space or account
isSpaceFollower
This checks if an account is following a particular space.
api.blockchain.isSpaceFollower(myAddress: AnyAccountId, spaceId: AnySpaceId): Promise<boolean>
Example:
const isFollower = await api.blockchain.isSpaceFollower('3osmnRNnrcScHsgkTJH1xyBF5kGjpbWHsGrqM31BJpy4vwn8', idToBn('1'))
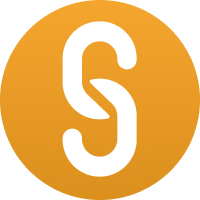
isAccountFollower
This checks if an account is following a particular account.
api.blockchain.isAccountFollower(myAddress: AnyAccountId, followedAddress: AnyAccountId): Promise<boolean>
const isFollower = await api.blockchain.isAccountFollower('3osmnRNnrcScHsgkTJH1xyBF5kGjpbWHsGrqM31BJpy4vwn8', idToBn('1'))
🆃 AnyAccountId: AccountId | string
🅸 AnySpaceId: SpaceId | BN
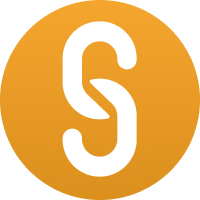
Find and load a list of space followers
spaceIdsFollowedByAccount
Get an array of space IDs that an account is following.
api.blockchain.spaceIdsFollowedByAccount(account: AccountId)
Example:
import { bnsToIds } from '@subsocial/utils'
const res = await api.blockchain.spaceIdsFollowedByAccount('3osmnRNnrcScHsgkTJH1xyBF5kGjpbWHsGrqM31BJpy4vwn8')
const followedSpaceIds = bnsToIds(res) // array of Space ids
spaceFollowers
Get an array of account IDs that follow a space.
substrateApi.query.spaceFollows.spaceFollowers(spaceId: SpaceId)
Example:
const followersBySpaceId = await substrateApi.query.spaceFollows.spaceFollowers('1')
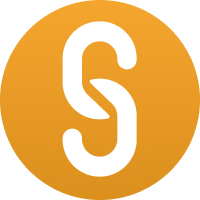
Find and load the list of followers and followings
accountFollowers
Get an array of account IDs that follow an account.
const substrateApi = await api.substrateApi
const res = await substrateApi.query.accountFollows.accountFollowers('3osmnRNnrcScHsgkTJH1xyBF5kGjpbWHsGrqM31BJpy4vwn8')
const accountFollowersIds = res
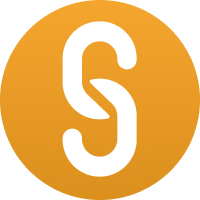
accountsFollowedByAccount
Get an array of account IDs that an account is following.
const substrateApi = await api.substrateApi
const res = await substrateApi.query.accountFollows.accountsFollowedByAccount('3osmnRNnrcScHsgkTJH1xyBF5kGjpbWHsGrqM31BJpy4vwn8')
const accountsFollowedByAccountIds = res
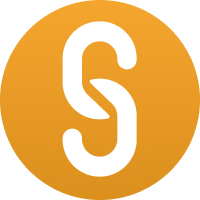