Create and update Posts
This section covers how to create and update posts on the Subsocial blockchain.
Create A Post
api.substrateApi.tx.posts.createPost(spaceIdOpt, extension, content)
Params | Description |
---|---|
spaceId | The space where a post will be published. |
extension | A kind of post. It can be a regular post, a shared post or a comment. |
content | IpfsContent is a function that returns a substrate like implementation for enum { IPFS: "CID of your content"}. |
🆃 PostExtensionEnum: RegularPost | Comment | SharedPost
Examples:
A Regular Post
import { IpfsContent } from "@subsocial/api/substrate/wrappers"
...
const cid = await api.ipfs.saveContent({
title: "What is Subsocial?",
image: "QmcWWpR176oFao49jrLHUoH3R9MCziE5d77fdD8qdoiinx",
tags: [ 'Hello world', 'FAQ' ],
body: 'Subsocial is an open protocol for decentralized social networks and marketplaces. It`s built with Substrate and IPFS.'
})
const tx = substrateApi.tx.posts.createPost('1', { RegularPost: null}, IpfsContent(cid))
...
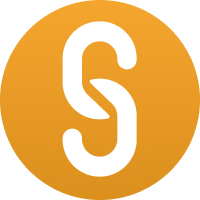
A Shared Post
import { IpfsContent } from "@subsocial/api/substrate/wrappers"
...
const cid = await ipfs.saveContent({
body: 'Keep up the good work!'
})
const tx = substrateApi.tx.posts.createPost('1', { SharedPost: '1'}, IpfsContent(cid))
...
}
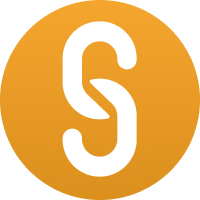
Update A Post
substrateApi.tx.posts.updatePost(postId: AnyPostId, update: PostUpdateType)
Params | Description |
---|---|
postId | The ID of the current space. |
update | The fields available to be updated. |
Update properties
Properties | Description |
---|---|
spaceId? | If a new spaceId is provided, it will move this post to the new space. |
content? | IpfsContent is a function that returns a substrate like implementation for enum { IPFS: "CID of your content"}. |
hidden? | boolean, if post is hidden from other users. |
Example:
import {
IpfsContent,
OptionBool,
} from "@subsocial/api/substrate/wrappers"
...
const cid = await api.ipfs.saveContent({
title: "What is Subsocial?",
image: "QmcWWpR176oFao49jrLHUoH3R9MCziE5d77fdD8qdoiinx",
tags: ['Hello world', 'FAQ', 'Subsoical'], //updated field
body: 'Subsocial is an open protocol for decentralized social networks and marketplaces. It`s built with Substrate and IPFS.'
})
const update = {
content: IpfsContent(cid),
hidden: new OptionBool(true),
}
const tx = substrateApi.tx.posts.updatePost('1', update)
...
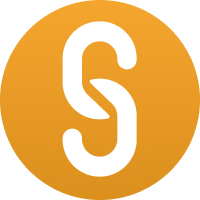