Create And Update Spaces
This section covers how to create and update spaces on the Subsocial blockchain.
Create A Space
substrateApi.tx.spaces.createSpace(content, permissionsOpt)
Params | Description |
---|---|
content | IpfsContent is a function that returns a substrate like implementation for enum { IPFS: "CID of your content"}. |
permissions | advanced options. |
Example:
import { IpfsContent } from "@subsocial/api/substrate/wrappers"
...
const cid = await api.ipfs.saveContent({
about: 'Subsocial is an open protocol for decentralized social networks and marketplaces. It`s built with Substrate and IPFS',
image: 'Qmasp4JHhQWPkEpXLHFhMAQieAH1wtfVRNHWZ5snhfFeBe', // ipfsImageCid = await api.subsocial.ipfs.saveFile(file)
name: 'Subsocial',
tags: [ 'subsocial' ]
})
const tx = substrateApi.tx.spaces.createSpace(
IpfsContent(cid),
null
)
...
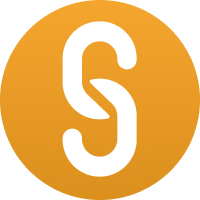
Update A Space
substrateApi.tx.spaces.updateSpace(spaceId: AnySpaceId, update: SpaceUpdateType)
Params | Description |
---|---|
spaceId | ID of the current space. |
update | fields available to be updated. |
Update properties
Properties | Description |
---|---|
content? | IpfsContent is a function that returns a substrate like implementation for enum { IPFS: "CID of your content"}. |
hidden? | boolean, if space is hidden from other users. |
permissions? | advance opt. |
Example:
import {
IpfsContent,
OptionBool,
} from "@subsocial/api/substrate/wrappers"
...
const cid = await api.ipfs.saveContent({
about: 'Subsocial is an open protocol for decentralized social networks and marketplaces. It`s built with Substrate and IPFS',
image: 'Qmasp4JHhQWPkEpXLHFhMAQieAH1wtfVRNHWZ5snhfFeBe',
name: 'Subsocial updated', // updated field
tags: ['subsocial']
})
const update = {
content: IpfsContent(cid),
hidden: new OptionBool(true),
}
const tx = substrateApi.tx.spaces.updateSpace('1', update)
...
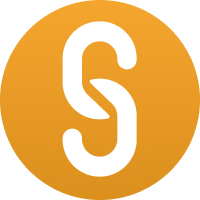
Space Permissions
Note: Blockchains by nature are public and transparent, which means that anyone can read any data you store in these spaces. To hide it from people, you can to encrypt the data from your end.
Type | Description |
---|---|
none | Read-Only Content |
everyone | Everyone can write. |
follower | Only the space followers can write. |
spaceOwner | Only the space owner can write. |
Writing includes both updating the space data and adding new posts to a particular space.
The way you can set permissions is:
const tx = substrateApi.tx.spaces.createSpace(IpfsContent(cid), {follower: true})
🆃 SpaceUpdateType: { content: SpaceContent, handle: string, hidden: Option<bool>, permissions: Option<Option<SpacePermissions (none, everyone, follower, space_owner)>> }